December 4, 2022
Read Time: 4 mins 21 secs
Word Count: 1034
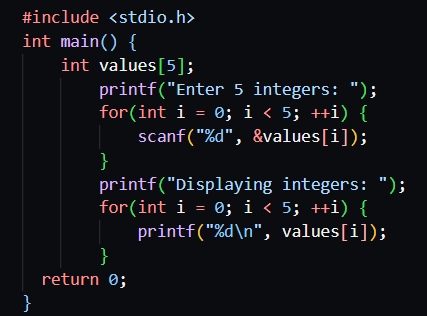
What are Arrays
An array is defined as a homogeneous, contiguous block of memory. An array is a collection of variables with the same data type and name. It is a data structure consisting of related data items. Array elements are stored in sequential memory and contiguous locations. Arrays are "static" entities in that they remain the same size throughout program execution. Any one of these elements may be referred to by giving the array’s name followed by the position number of the particular element in square brackets ([]).
Arrays can be classified as:
Single-Dimensional Array (1 row only)
Multi-Dimensional Array (rows and columns).
The Purpose of Arrays
Primitive variables store one value at a time, whereas arrays may store multiple values or data items at a time. Arrays are commonly used in computer programs to organize data so that a related set of values can be easily sorted or searched. E.g., a search engine may use an array to store Web pages found in a search performed by the user. When displaying the results, the program will output one element of the array at a time.
Initializing Arrays
The first element in every array is the zeroth element. An array name, like other identifiers, can contain only letters, digits, and underscores and cannot begin with a digit. The elements of an array can be initialized when the array is defined by following the definition with an equal sign and braces containing a comma-separated list of initializers. ({1, 2, 3, 4}) If there are fewer initializers than the size of the array, the remaining elements are initialized to zero. If the array size is omitted from a definition with an initializer list, the number of elements in the array will be the number of elements in the initializer list. The #define preprocessor directive can be used to define a symbolic constant. When the program is preprocessed, all occurrences of the symbolic constant are replaced with the replacement text. e.g., #define SIZE 10
Syntax
int n[8] = {5, 2, 3, 10, 9};
[5] [2] [3] [10] [9] [0] [0] [0]
Declares the first 5 and places 0 in the remainingAccessing an array
An element is accessed by indexing (numbering) the array name. This is done by placing the index of the element within square brackets after the name of the array. The array index starts at 0, so the index ranges from 0 to arraySize - 1 The most efficient way to access all the elements in an array is to use a loop to move through each index of the array. It is very common to see arrays and for loops used together. This is probably because for loops are used to do a specific number of loops, and arrays are always of a specific length. This makes for loops ideal for stepping through the elements of an array. C has no array bounds checking to prevent a program from referring to an element that does not exist Thus, an executing program can "walk off" the end of an array without warning. You should ensure that all array references remain within the bounds of the array. The most efficient way to access all the elements in an array is to use a loop to move through each index of the array. It is very common to see arrays and for loops used together. This is probably because for loops are used to do a specific number of loops, and arrays are always of a specific length. This makes for loops ideal for stepping through the elements of an array.
Arrays and Functions
To pass an array argument to a function, specify the array’s name without the square brackets. Unlike char arrays that contain strings, other array types do not have a special terminator. For this reason, the size of an array is passed to a function so that the function can process the proper number of elements. Although entire arrays are passed by reference, individual array elements are passed by value, exactly as simple variables are. Such simple single pieces of data (such as individual ints, floats, and chars) are called scalars. To pass an element of an array to a function, use the subscripted name of the array element as an argument in the function call.
Multi Dimensional Array
An array containing more than one level or dimension is referred to as multi-dimensional. For instance, a 2D array, often known as a two-dimensional array, is a matrix of rows and columns . An array of arrays of arrays is therefore created.
The simplest form of a multidimensional array is a two-dimensional array. The first index of the array represents rows of the table, and the second index represents columns of the table.
[datatype] [arrayName] [rows] [columns];
[int] [cars] [2] [3];
Calculate the size of a multidimensional array by multiplying the sizes of all the dimensions. The array's size can be calculated as the number of rows multiplied by (x) the number of columns.
The size of the array above is 2 * 3, which is equal to 12 elements in the array.
Initializing Multi Dimensional Array
[int] [cars] [2] [3] = {1,2,3,4,5};
If the braces around each sub-list are removed from the array’s initializer list, the compiler initializes the elements of the first row followed by the elements of the second row. The definition above provides five initializers. The initializers are assigned to the first row, then the second row. Any elements that do not have an explicit initializer are initialized to zero automatically, so b[1] [2] is initialized to 0.
Accessing Multi Dimensional Array
To manipulate a multi-dimensional array, a nested for loop is needed. A loop is a set of instructions that are repeatedly carried out until a specific condition is met. Typically, a certain action is taken, such as receiving and modifying a piece of data, and then a condition is verified, such as determining whether a counter has reached a predetermined value. A loop inside the body of the outer loop is known as a nested loop. Any sort of loop, like a while loop or a for loop, can be used as the inner or outer loop.