Introduction to Object Oriented Programming
December 15, 2022
Read Time: 7 mins 10 secs
Word Count: 1707
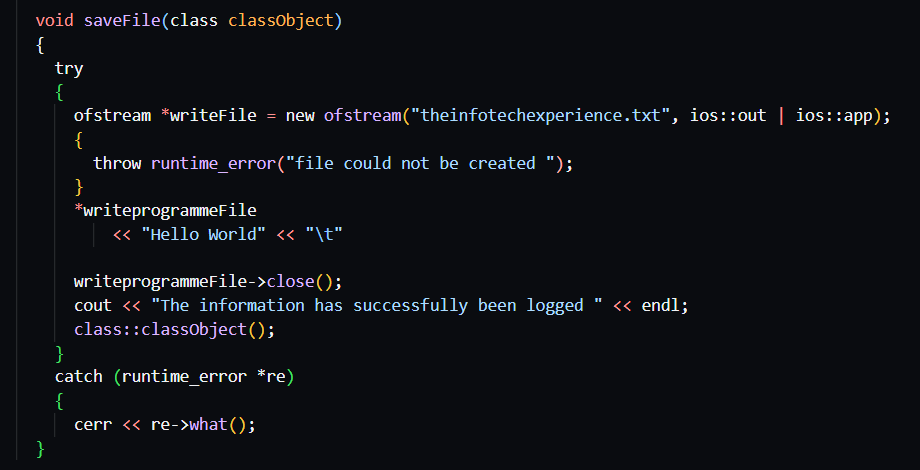
What is a programming language
A programming language is a notational system used to describe computation in both machine-
readable and human-readable form. Historically, there have been three types of
programming languages: Machine languages, Assembly Languages and High-level Languages.
Machine Languages
Machine language is the only language the computer can read or understand directly. This language is hardware dependent. Hardware dependent means the language the machine language is written in should be designed to the specific hardware device. Machine language is made of binary integers, is read by the CPU of the computer, and looks like lengthy stream of ones and zeros.
Assembly Languages
Assembly language, commonly abbreviated as ASM or asm, first appeared in 1947. Assembly language is used to directly alter hardware, access specific processor instructions, or assess serious performance problems. These languages are also utilized for time-sensitive operations like high frequency trading to take full advantage of their speed advantage over high level languages. It speaks in a coded, symbolic manner. The use of assembly language enables direct hardware communication. This language is mostly focused on computer architecture, and it can identify certain processor types and how they differ for various CPUs.The language uses mnemonics to represent machine language instructions. Symbolic labels are used for memory addresses. It must be translated to machine language to be executed on the target machine and the language type encourages spaghetti code.
Data Types that appear in Assembly language are
- BYTE- A BYTE is an 8-bit unsigned integer
- SBYTE - A BYTE is an 8-bit unsigned integer
- WORD - A WORD is a 16-bit unsigned integer
- SWORD - A SWORD is a 16-bit unsigned integer
- DWORD - A DWORD is a 32-bit unsigned integer
- SDWORD - A SDWORD is a 16-bit unsigned integer
- FWORD A FWORD is a 48-bit unsigned integer
- QWORD - A QWORD is a 64-bit unsigned integer.
High-level Languages
High-level Languages programs written with instructions closely
resembling natural languages and mathematics. A programming language that has a strong separation from the specifics of the machine is considered high-level. High-level languages have the benefit of being much simpler to build programs in than low-level languages. A human can understand a program much more easily since many high-level languages include common command terms like IF, ELSE, and FOR. High-level languages can be used virtually anywhere.
High-level language's source code is machine independent.
High-level languages has to be translated to machine language to be
executed.
Popular High-Level Languages are:
- JavaScript.
- Python.
- C++
- C#
- Java
What is a programming language paradigm
A programming language paradigm is a particular style of programming in which the concepts and abstractions differ. Paradigms such as Imperative, Declarative and Object-Oriented
Imperative Programming Paradigm
Imperative Programming describes computation as a series of steps,
that is, as an algorithm, hence also called
algorithmic programming paradigm. It is sometimes called Procedural Programming. Formally, Procedural Programming is a type
imperative programming in which the
computational steps are placed in procedures. Characteristics of the paradigms Imperative Programming Paradigm Modifies state e.g. through variable assignment. Early machine languages were the first imperative programming languages. By extension assembly language was
imperative programming. Early high-level languages were also based on the imperative programming language paradigm.
Declarative Programming Paradigm
The declarative programming paradigm involves stating what computation is to be done without specifying how it is to be done. Declarative language lets programmers realize what to observe so that they can subsequently come to crucial conclusions on their own, rather than telling them what to do, which fosters inferential thinking. It offers students the freedom to operate, which facilitates spontaneity and independence. Therefore because developers express what they would like the program to achieve exactly rather than explaining how to achieve it, declarative programming is simple to read. Declarative programming reduces data mutability, which enhances program security because immutable data structures are generally less error-prone. It Includes functional and logical programing. The paradigm lacks side-effects (functions or relations do not modify values in memory but may transform the values into new ones).
Object-Oriented Programming Paradigm
The object-oriented programming paradigm Focuses on on “objects” - which are collections
of data items and the operations that can be
performed on them. In this paradigm Messages can be sent to an object to tell it to
perform a particular operation or method, operations belonging to an object are called
methods and Data elements of objects are referred to as attributes.
The Object-oriented programming paradigm has four categories. Abstraction, Encapsulation, Inheritance and Polymorphism.
The object-oriented programming notion of abstraction "shows" only necessary properties and "hides" redundant data. Abstraction serves the primary function of sheltering users from inconsequential details. The term encapsulation refers to the grouping together of methods that operate on data and the data itself. Inheritance essentially enables code reuse.
Consider a scenario in which you have an existing class and you wish to create a brand-new one that uses the features of the old class but adds new capabilities.
The class from which a subclass might be descended is known as the superclass or parent class, and the classes that are derived from existing classes are known as subclasses or child classes.
Polymorphism is the ability of a superclass reference to change from referring to superclass objects to subclass objects.
Advantages of Object Oriented Programming
- Makes Code Maintenance Easier
- Reduces The Cost of development
- Allows Programmer to Reuse Code
- Adaptable Code
- Security of Code
- Software that is object-oriented is easier to maintain in terms of the code. Because of the design's flexibility, a component of the system may be upgraded in the case of issues without necessitating major changes. You may also alter already-existing items to produce new ones.
This feature would be useful for any programming language since it saves users from having to duplicate work in a plethora of ways. It is usually straightforward and simple to monitor and maintain the present codes by adding new modifications. It is straightforward to maintain and alter existing code since one may create new objects with just small modifications from existing ones.
-
It is feasible to reduce some of the direct expenses associated with systems, including maintenance and development, by using an object-oriented approach. Software reuse also lowers the cost of development. Object-oriented analysis and design typically take more time and effort, which lowers the overall development cost.
Since more time and effort is often placed into the article-specific assessment and plan, the overall cost of the improvement is decreased. Since object-oriented analysis and design typically take more time and effort, the development cost is typically lower.
-
One of the fundamental notions provided by object-oriented programming is the concept of inheritance. The attributes of a class may be handed down through inheritance, removing the need for duplication of effort. This eliminates the issues that arise from continuously writing the same code.
Because of the introduction of the concept of classes, the code block may be utilized as many times as needed in the application. A child class that employs the inheritance method inherits the fields and methods of the parent class. The accessible methods and values in the parent class may be easily changed.
- Polymorphism is the concept of adaptability. Polymorphism has the following benefits for developers: extensibility and simplicity. Polymorphism, which permits a piece of code to exist in more than one version, is one advantage of OOP. For example, if the setting or environment changes, you may behave differently.
Consider the following example. A person will act as a consumer in a market, as a student in a school, and as a son or daughter in a household. In this case, the same person shows different actions depending on the situation.
- These OOP programming languages preserve security while offering essential data to view by using data hiding and abstraction mechanisms to filter out restricted data from exposure.
Due to data abstraction in OOP languages, only a limited amount of data is displayed to the user, resulting in security advantages. While disclosing only the necessary quantity of data, the remaining data is not exposed. As a result, it provides for the retention of security. An additional advantage is that the notion of abstraction is used to conceal the complexity from other users and display the element's information based on the needs. It also aids in the avoidance of repeated code. Another idea given by OOP languages is encapsulation, which provides the protection of data in classes from being accessible by the system. All of the class's internal contents can be protected.
Sometimes a programming language may support multiple programming paradigms. Such as C++. Both procedural and object-oriented programs can be written in C++ and are supported by the C++ standard. This contrasts with C and Java, which only support procedural and object-oriented programming respectively.
Languages Belonging To Each Paradigm
Logical Paradigm:
Prolog, Godel
Functional Paradigm:
LISP, Haskel, ML, Miranda, APL
Object-Oriented Paradigm:
C++, Java, C#, SmallTalk, Simula, Eiffel, Ruby
Imperative/Algorithmic/Procedural Paradigm:
C, Pascal, BASIC, FORTRAN, COBOL, Algol, PL/1, Modula-3
What is an Object-oriented Analysis (OOA)
In object-oriented analysis (OOA), the analyst examines the written requirements and extracts the class objects and their elements and interactions. OOA can be performed by conducting a textual parse of the requirements. This textual parse is also called a noun-verb analysis. Formal methods such as those developed by Coad and Yourdon can be used. Nouns become classes, objects or attributes, while verbs become methods. Formal methods such as those developed by Coad and Yourdon can be used.
Coad and Yourdon's Six Characteristics for
Selecting an Item as an Object during OOA are
Retained Information, Needed Services, Multiple Attributes,Common Attributes,Common Operations, Essential Requirements
What is an Object-Oriented Design (OOD)
In object-oriented design, the system to be built is modeled. The classes and their relationships are usually modeled using the Unified Modeling Language (UML).UML is a diagrammatic language. Sometimes the analysis and design phases are combined into object-oriented analysis and design (OOAD)
Implementing the Design with an Object-Oriented Language
The model of the system in UML is implemented in the object-oriented programming language of choice by converting the objects and their relationships into corresponding appropriate programming language constructs. The code is then compiled into machine language or some other internal representation and executed on the target computer.